Java is a common programming language first released in 1995 by Sun Microsystems. Today, Java is open source and free for personal and developer use. It's one of the most popular programming languages due to its flexibility and design.
Other programming languages must have their code compiled for specific platforms, increasing the difficulty and cost of supporting software across many operating systems and CPU architectures. Java is different. It is described as write once, run anywhere (WORA) software. What makes Java unique is the code remains the same, but the runtime environment is platform-specific. For example, to run a Java application on a Mac, Linux device and Windows server, you simply write the code once and execute it in Java Virtual Machines (JVM) created for each operating system (OS). If the platform has a JVM, it can run the code. That includes regular computers but also mobile and embedded devices.
Common Java attributes include:
- Multiplatform
- Fast
- Secure
- Reliable
- Easy to learn
Java has strong documentation and an even stronger community supporting it. And Java software is everywhere, from games to internet of things (IoT) devices to the cloud and mobile systems. Java developers have access to a wide variety of development environments.
If you're considering a career in application development and are curious about Java, this article will provide crucial information you need to know.
Key Features of Java
Like other mature programming languages, Java has an extensive list of libraries. These libraries consist of ready-made tested code (Java classes), allowing developers to be more efficient and include greater functionality.
Here are a few common Java libraries:
- Java Standard Library: Including lang and math
- Google-json: Allowing conversions between Java objects and JSON files
- Log5j: A library of logging features for Java
- JFreeChart: A library for displaying graphs and charts
You may already be familiar with the concept of libraries from other programming languages, such as Python.
Java programming relies on three main components:
- Java Development Kit (JDK): Development environment
- Java Runtime Environment (JRE): Execution environment
- Java Virtual Machine (JVM): Runtime environment
The JDK allows Java developers to create their applications. It is platform-specific (Linux, macOS, Windows, etc.) and contains the compiler, debugger and other components necessary for programming. It also includes the Java Runtime Environment and Java Virtual Machine. The JDK is the Java implementation of a Software Development Kit (SDK).
The Java Runtime Environment is an execution point for Java code. It contains the necessary libraries and other components for running Java code. It also includes the JVM.
The JVM is an environment for an instance of Java code executing. It loads and runs the bytecode generated by Java. JVMs are platform-specific, which is what allows for Java's portability. Java code executes in the JVM, so if your system can run a JVM, it can run Java. For the most part, that means Linux, macOS, Windows and Solaris platforms.
While you’ll likely begin with the Standard Edition, there are three Java editions:
- Java Platform, Standard Edition: Created for workstations and general functionality
- Java Platform, Enterprise Edition: Created for larger distributed infrastructures
- Java Platform, Micro Edition: Designed for platforms with limited resources like IoT devices
Java Integrated Design Environments
It's important to have the right tools for the job, and if you're working with Java, that means a good integrated development environment (IDE) or at least a text editor.
Most IDEs offer similar features:
- Editors with syntax checking and autocompletion
- Support for multiple programming languages
- Build tools
- Debugging capabilities
- Source and version control (or integration with services that provide these)
With Java's long history, programmers have a lot of choices.
Eclipse
Eclipse is a great Java IDE to get started with. It's very popular and supports many languages in addition to Java. You can install it on your Mac, Linux or Windows systems, which is handy in cross-platform environments. It has a strong support community, which has developed many plugins over the years. Be aware that Eclipse can be resource-intensive. Eclipse is available for free.
IntelliJ IDEA
A competitor to Eclipse, IntelliJ offers robust features and excellent user satisfaction ratings. It's feature-rich, customizable and integrates with various other developer tools. The IDE runs on Windows, Linux and macOS. It's available in a free community edition and a more robust paid version.
NetBeans
Another solid choice for Java development is NetBeans. It's very popular, easy to use and cross-platform. Like Eclipse, however, NetBeans can be resource-intensive. It is also free.
BlueJ
BlueJ is a relatively simple IDE, which makes it a great choice for beginners. It runs on Mac, Windows and Linux operating systems. It's best used in smaller deployments or learning labs, as it only supports Java. You can download and use BlueJ for free.
Text Editors
There's nothing wrong with the good ole text editor when it comes to simple Java projects. Something like Vim or Notepad++ would be a perfect way to get started with a few lines of code. These sorts of text editors often have Java capabilities or can easily be extended for Java syntax checking, highlighting, etc.
Common Java Concepts
Most programming languages have various things in common. Java supports variables, loops, strings and other components that comprise a standard set of programming capabilities. Java is case-sensitive, so keep that in mind when naming files or setting classes.
Lines of Java code are contained in classes. Class names begin with an uppercase character and the java file extension. You'll see an example in the next section named Main.java.
Like Python, Bash scripts, JavaScript and other programming/scripting languages, Java uses comments to allow developers to insert documentation, examples or other explanations into the code. Comments are delineated with the // characters.
You may declare variables to store values for later use, or you may choose to insert for or while loops to repeat sections of code.
When to Use Java
Java pervades almost every industry, making Java developers very popular in the fields of mobile app development, game deployment, big data analysis and machine learning. Java spans multiple industries, from finance to science to education. There always seems to be plenty of room in the workforce for more Java developers, whether they are new to the field or experienced with other programming languages.
Some Java Project Examples
When learning a programming language, it's good to have a goal in mind. It can be challenging to read about abstract concepts like variables or loops. A project gives you a way to be enthusiastic about what you're learning.
Search online for interesting Java projects, or consider some of the following ideas:
- Build a calculator
- Build a currency conversion tool
- Construct a digital clock
- Create a tick-tack-toe game
There are hundreds more choices. These challenges help you absorb and apply the concepts rather than just reading about them.
Get Started With Java
Some operating system versions ship with Java installed, and others don't. The installation process varies depending on whether you use Linux, macOS or Windows.
Type java -version to see whether Java is installed on your system:

Figure 1: Check the Java version on your system
Here is the traditional "Hello, World!" example to give you a chance to do a little Java programming.
I'll begin by writing a bit of Java code using the Vim text editor (my text editor of choice). Any text editor will do but don't use a word processor such as Microsoft Word or LibreWriter.
public class Main {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
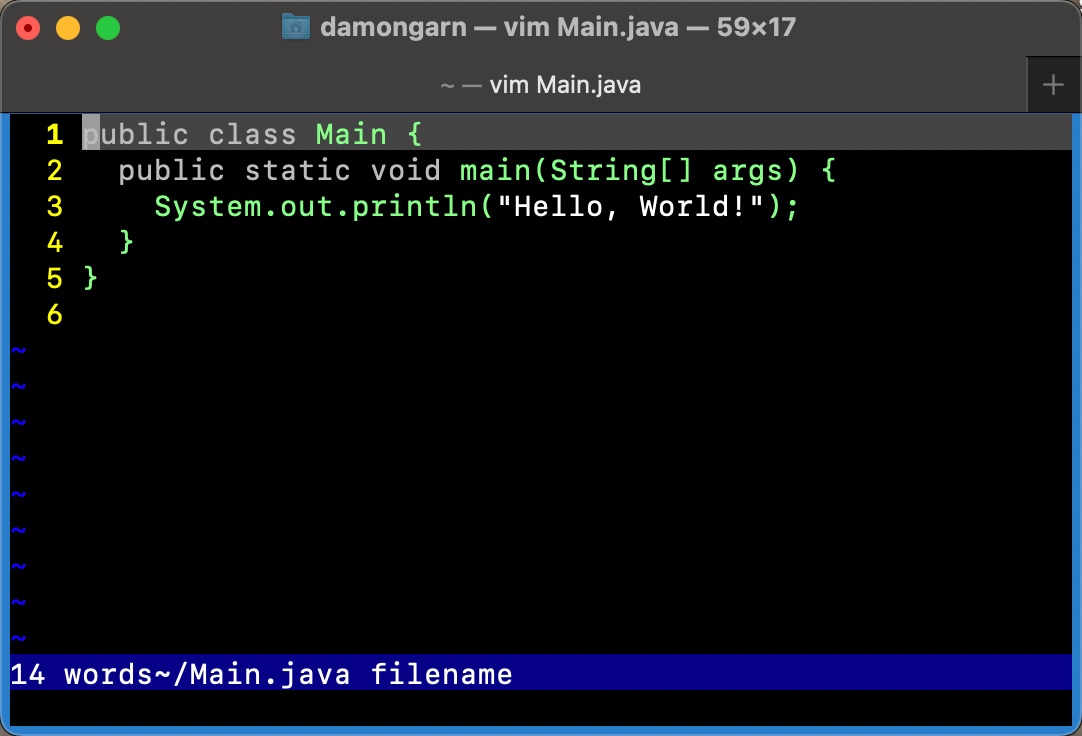
Figure 2: Java code to print "Hello, World!" to the screen
Save the file using the name Main.java. Note where the file is located (probably your home directory).
Next, you'll need to compile the code. Depending on your setup, you may be able to simply use the javac command. I needed to enter the full path to the OpenJDK compiler on my Mac.
/usr/local/opt/openjdk@8/bin/javac Main.java
There's no output from this command. If Java doesn't complain, then you've done it right.
Finally, run the program using the java command:
java Main
Java should greet you with a "Hello, World!" message.
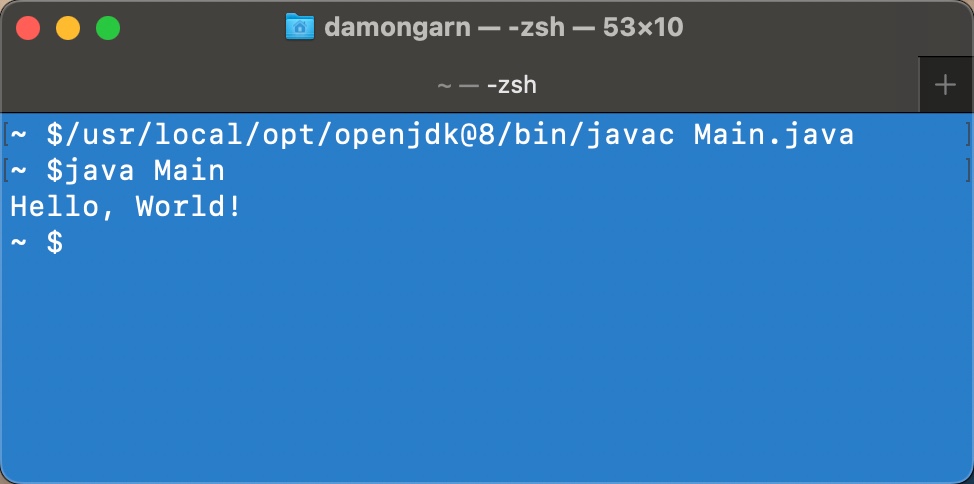
Figure 3: Results of the Java code
Welcome to Java!
Java Advantages and Disadvantages
Like any programming language, Java has advantages and disadvantages. Here are a few things to consider when thinking about learning Java.
The Advantages:
- Strong developer community for innovation and support
- Relatively simple structure
- Object oriented design
- Relatively secure
- Robust and reliable
- Long history with lots of deployed apps makes it a standard choice
- Portability/platform independence is higher than in other languages
The Disadvantages:
- Performance concerns because it is interpreted at runtime and therefore slower than other languages
- Syntax verbosity may make it more difficult to debug or learn
- Memory use is less efficient than programs written with C or C++
- Relatively poor backward compatibility with legacy code
One of the most important aspects of Java is the write once, run anywhere (WORA) portability. This is a critical differentiator from many other programming languages. The Java VM enables a different workflow from applications that must be compiled for specific platforms. Java is popular and common; it's a great career choice with a long future ahead of it. The strong community, long history and mature documentation also help.
My favorite way to use Java is the Minecraft Java Edition. I play Minecraft on my Mac, but the game uses a JVM between the code and the OS. It's a great example of Java's portability and write once, run anywhere approach.
Get the in-demand skills you need with CompTIA certifications and training solutions. Download the exam objectives to get started.